- Livewire Key Features That Make It Stand Out
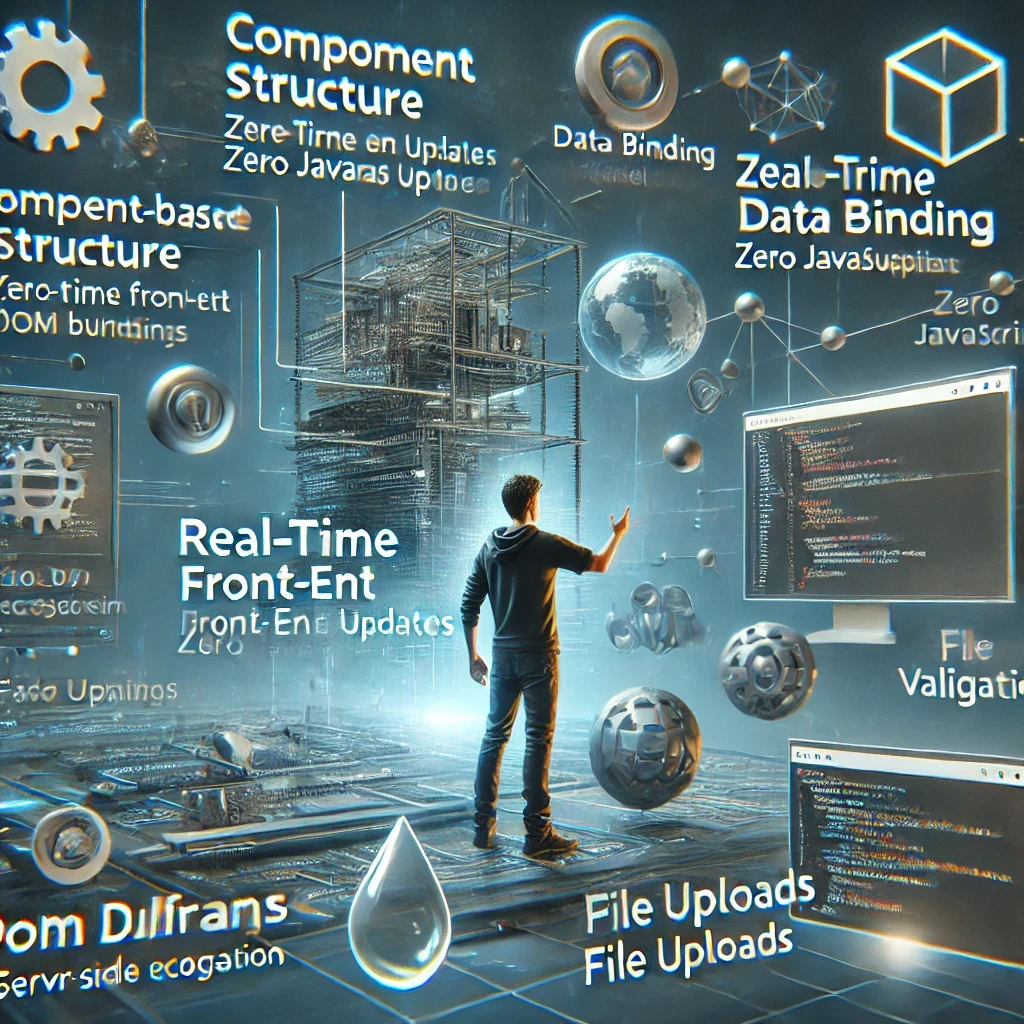
- 6 months ago
- 6 min read
Livewire is a full-stack framework for Laravel that allows you to build dynamic interfaces without leaving the comfort of Laravel. It provides the power of front-end interactivity using only PHP, eliminating the need to write significant amounts of JavaScript. For developers who are already familiar with Laravel, Livewire provides a seamless way to add advanced interactivity to your applications with ease. Below are some of the key features that make Livewire a standout choice for developers:
1. Component-Based Structure
Livewire revolves around the concept of reusable components. Each component is essentially a self-contained unit, containing both the server-side logic (PHP) and the front-end presentation (Blade templates). This structure enables clean separation of concerns while maintaining the simplicity of Laravel's Blade system. You can create and organize components to handle specific tasks like forms, tables, or any other UI interaction.
Example:
A simple component to handle user input could look like this:
class UserInput extends Component { public $name = ''; public function updatedName($value) { $this->name = strtoupper($value); } public function render() { return view('livewire.user-input'); } }
This component automatically listens for changes and updates the view dynamically.
2. Real-Time Front-End Updates
With Livewire, you can easily create real-time updates in your application. It uses WebSockets or AJAX to communicate with the backend whenever a user interacts with the page. This allows components to update without requiring a full page reload, making your application feel more like a Single Page Application (SPA) but without the overhead of managing complex JavaScript frameworks like Vue or React.
Example Use Case:
For instance, a shopping cart can update instantly as users add or remove items, providing immediate feedback without a page refresh. This makes your application faster and more responsive.
3. Zero JavaScript
One of Livewire's most attractive features is the ability to handle complex interactions without needing any JavaScript. While under the hood Livewire uses AJAX and WebSockets, you as the developer don't need to write a single line of JavaScript. The framework abstracts these complexities and provides a clean, declarative syntax.
Example:
If you want a button to trigger a backend function, you can simply write:
<button wire:click="submitOrder">Submit</button>
Livewire will handle the server communication, and you can focus solely on PHP.
4. Data Binding
Livewire's data binding allows you to synchronize data between the front-end and back-end effortlessly. It supports both simple data binding (e.g., updating an input field) and advanced two-way binding where changes in the view automatically reflect on the server-side variable.
Example:
<input type="text" wire:model="name">
In this case, the $name variable on the server side will automatically update whenever the user changes the input field.
5. Laravel Ecosystem Integration
Livewire is tightly integrated with Laravel, which means you get access to the entire Laravel ecosystem—ORM (Eloquent), validation, routing, and more—within your Livewire components. This allows developers to easily manage application logic, interact with databases, and leverage all Laravel's powerful tools in their Livewire components.
Example:
For validation, you can simply use Laravel’s validation system within Livewire components:
$this->validate([ 'email' => 'required|email', 'password' => 'required|min:6', ]);
6. Automatic DOM Diffing
Livewire automatically handles DOM diffing, which means only the parts of the page that need updating are re-rendered. This results in faster, more efficient updates, reducing unnecessary server requests and data transfers. It makes Livewire a very performant solution for dynamic web applications.
7. File Uploads Made Easy
Handling file uploads with Livewire is incredibly simple. You don't need to worry about JavaScript libraries for file handling. Livewire has built-in support for file uploads with robust validation, progress indicators, and real-time updates.
Example:
<input type="file" wire:model="photo">
On the server side, Livewire handles the file upload and validation processes, simplifying what would typically be a multi-step operation in JavaScript.
8. Lifecycle Hooks
Like JavaScript frameworks such as Vue and React, Livewire provides lifecycle hooks that let you run code at specific points in a component's life cycle. You can use these hooks to listen for when a component is mounted, updated, or destroyed, enabling more control over how your components behave.
Example:
public function mount() { // Code to run when the component is initialized }
This gives you flexibility to manage component state and interaction effectively.
9. Server-Side Validation
Livewire allows for server-side form validation, removing the need for handling validation logic in JavaScript. You can perform validation in your PHP component and instantly reflect validation errors in the UI, keeping the entire flow seamless.
Example:
$this->validate([ 'name' => 'required|string|max:255', 'email' => 'required|email', ]);
Any validation errors will automatically be displayed in the Blade template, making the user experience smooth and efficient.
10. Loading States
With Livewire, it's easy to show loading states when making a request to the server. This helps improve user experience by informing users that something is happening in the background.
Example:
<button wire:click="submitOrder" wire:loading.attr="disabled">Submit</button>
Here, the submit button will be disabled while the server is processing the request.
Conclusion
Livewire bridges the gap between back-end development and front-end interactivity by providing a powerful, simple, and flexible framework for Laravel developers. With its component-based structure, real-time updates, zero JavaScript requirement, and seamless Laravel integration, Livewire empowers developers to create dynamic and responsive web applications quickly. Whether you're building small components or full-scale applications, Livewire offers the tools to make the development process efficient and enjoyable.
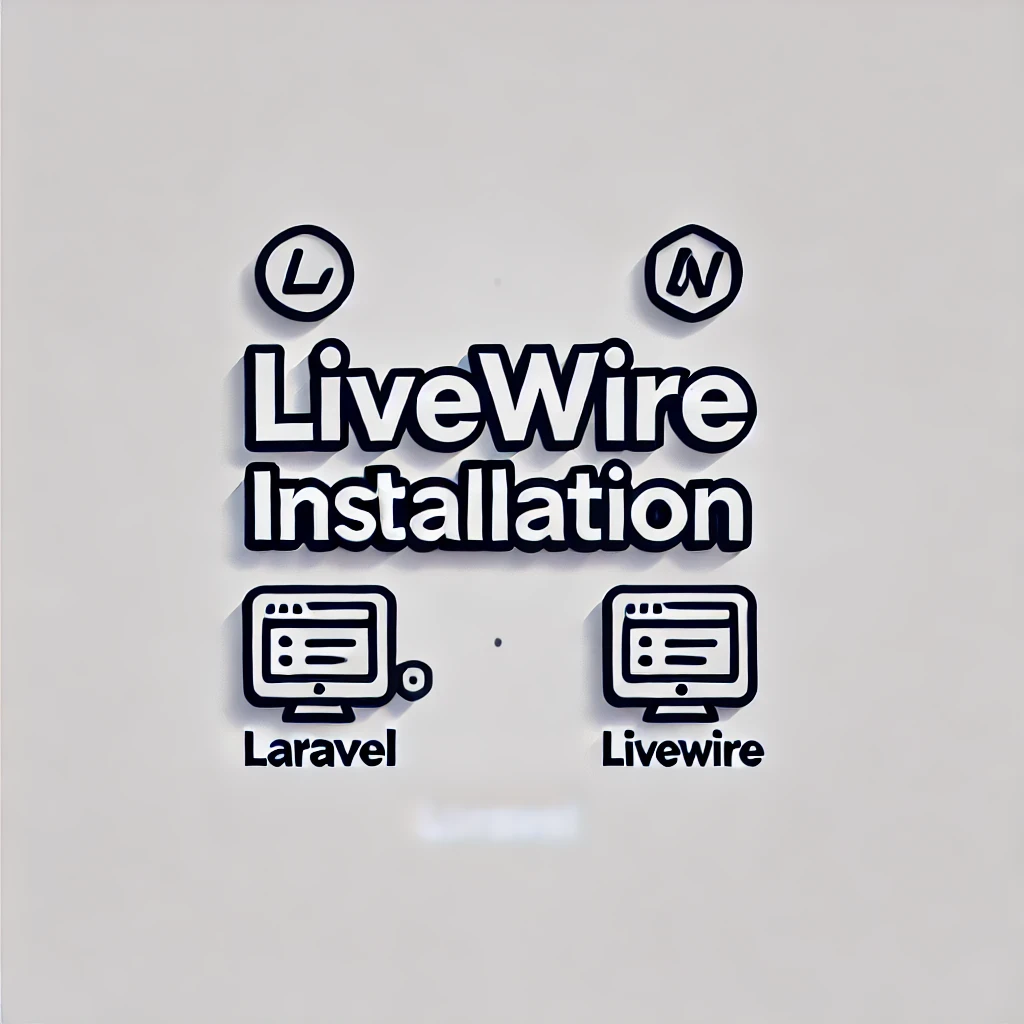
Step-by-step tutorial to install Livewire
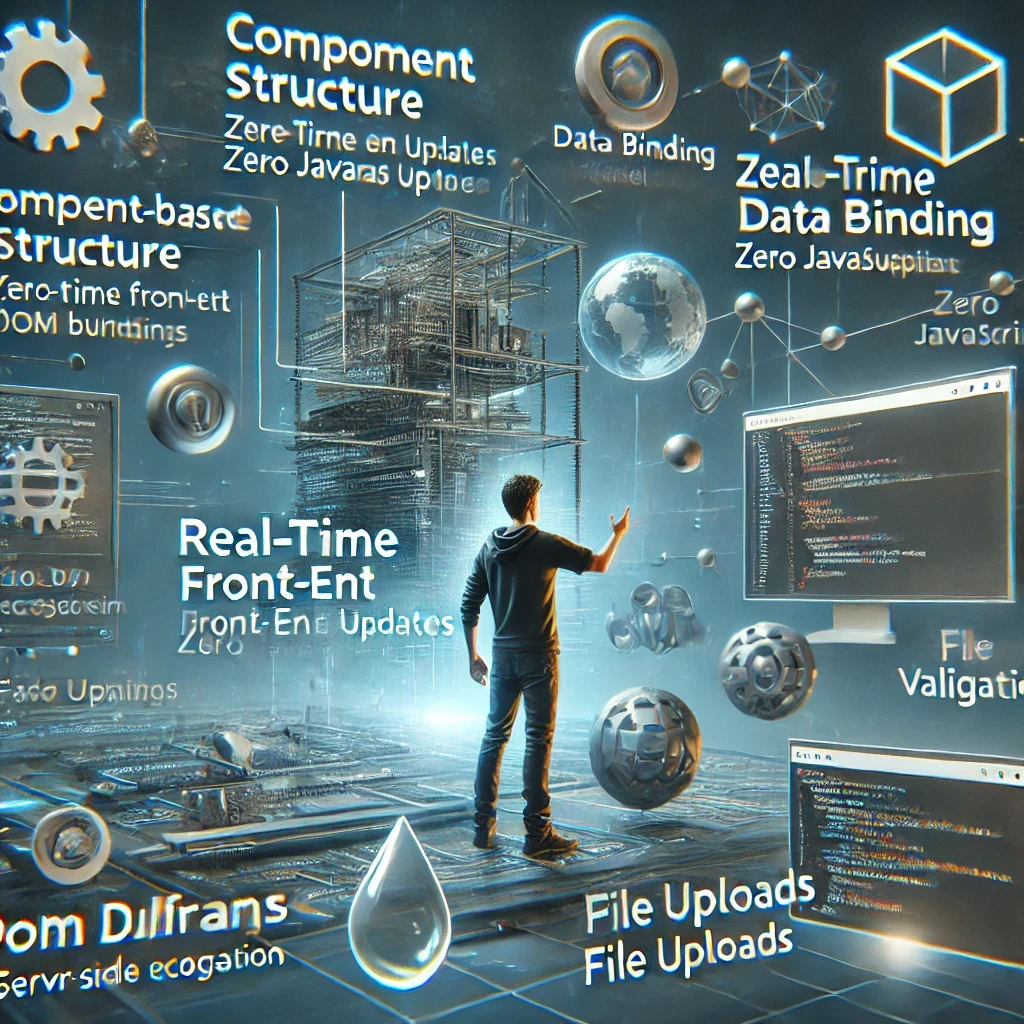
Livewire Key Features That Make It Stand Out
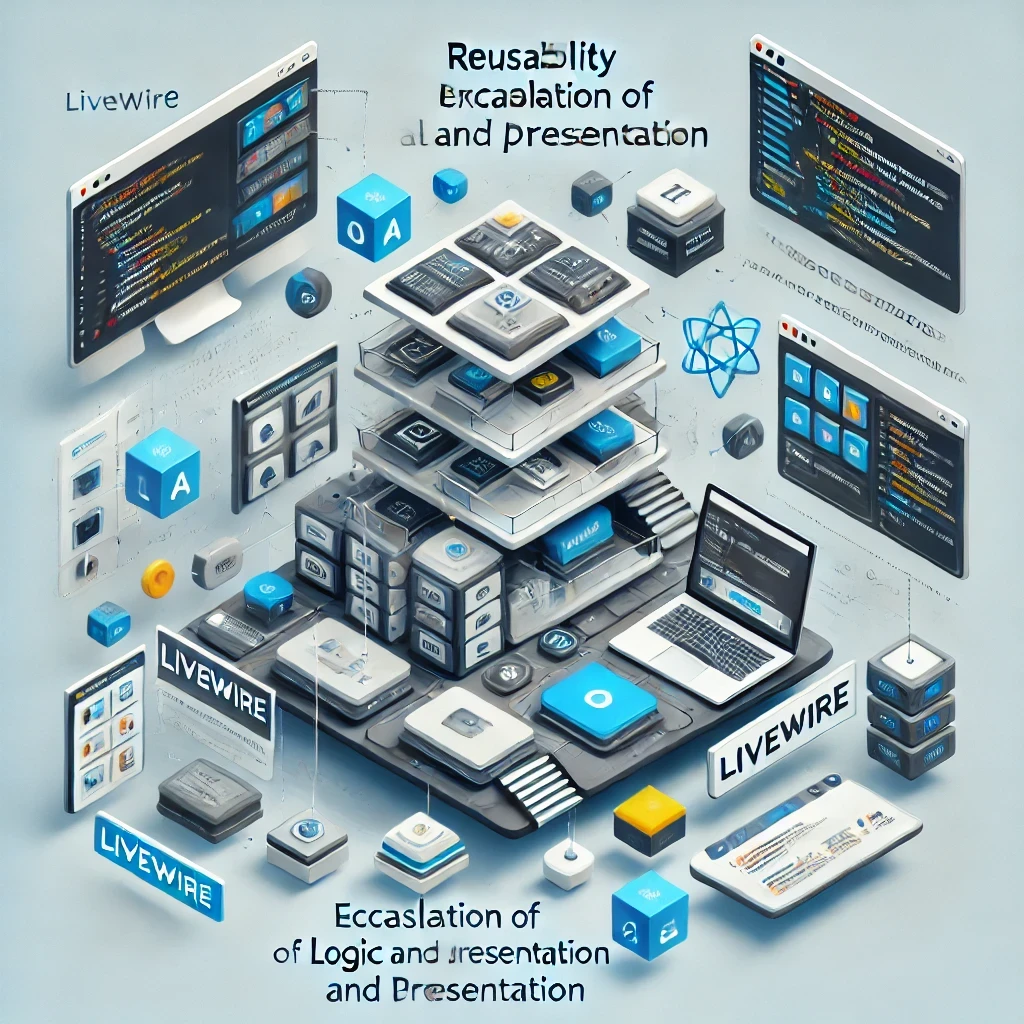
What is Component-Based Architecture ?
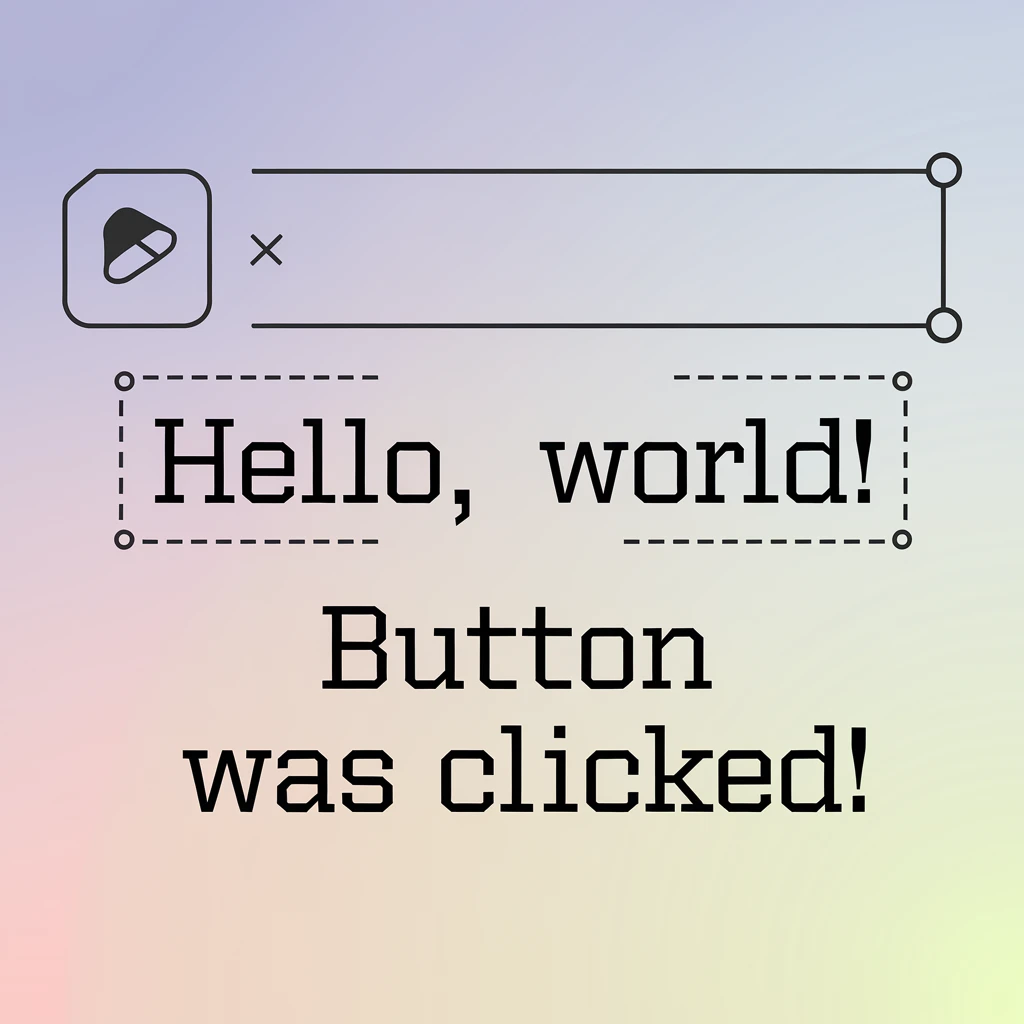
Real-Time Front-End Updates in Livewire
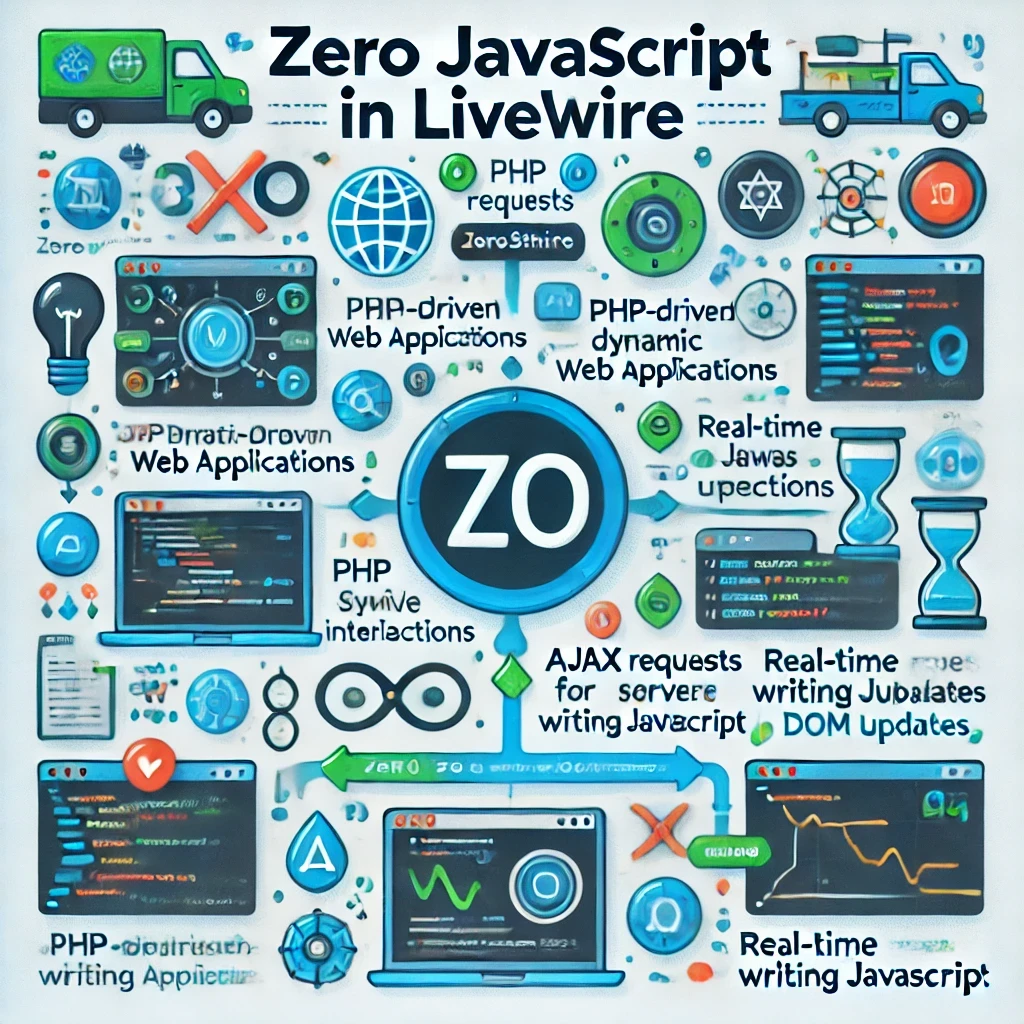
Zero JavaScript in Livewire Revolutionizing Laravel Development
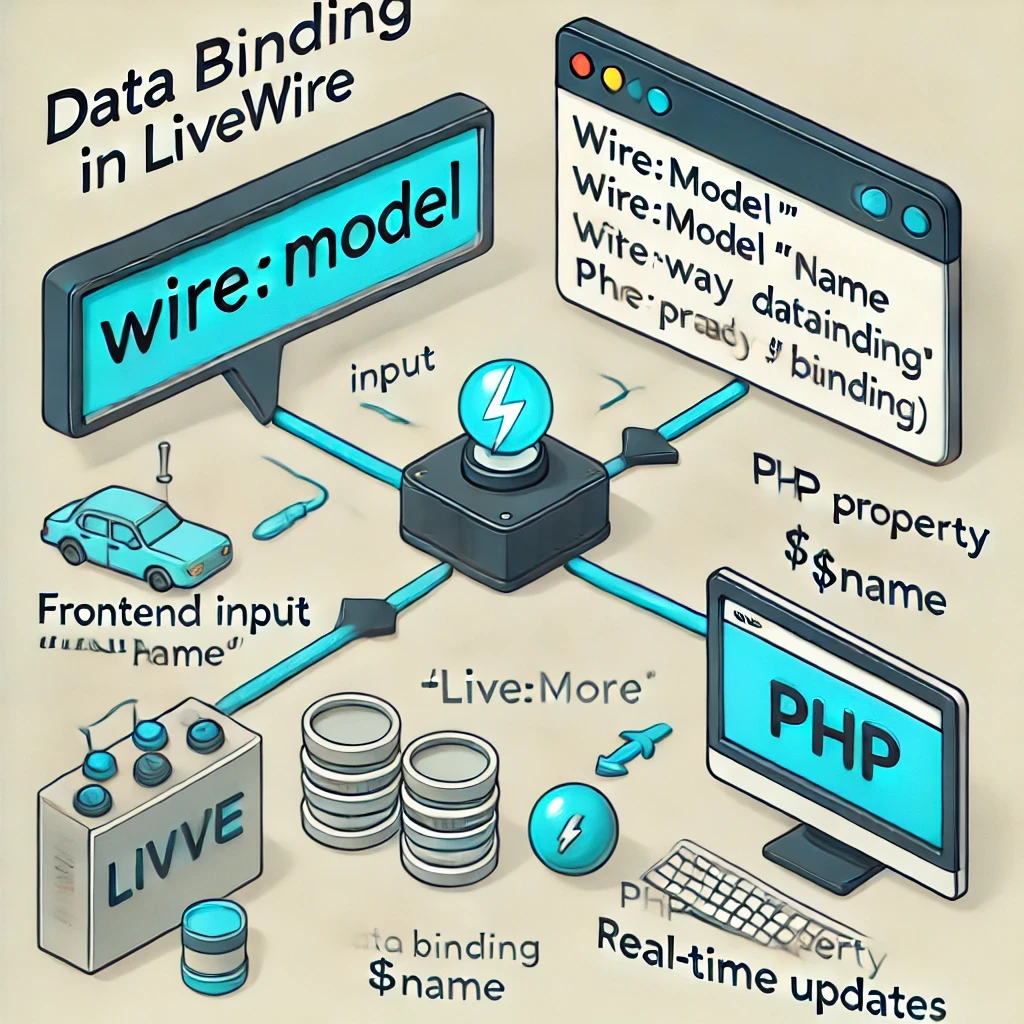
Data Binding in Livewire A Seamless Way to Sync Data
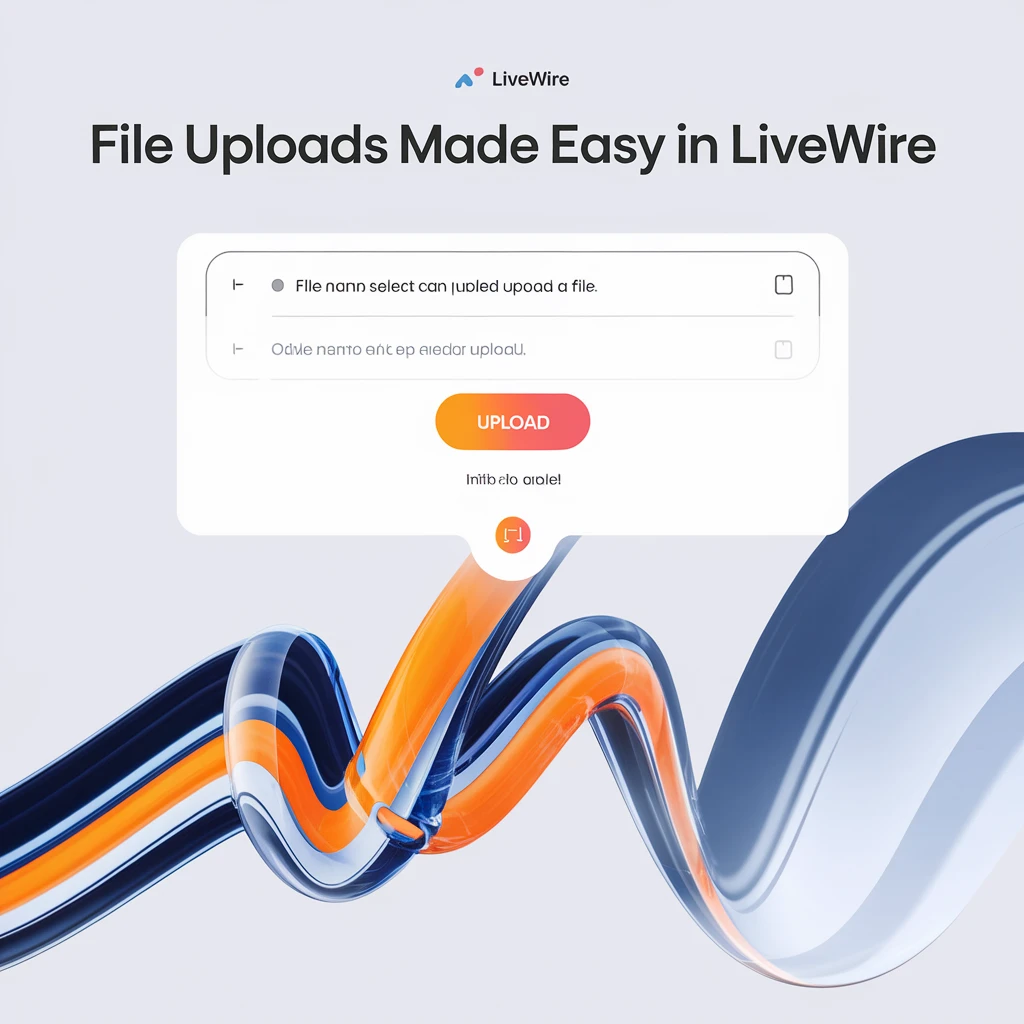
File Uploads Made Easy in Livewire
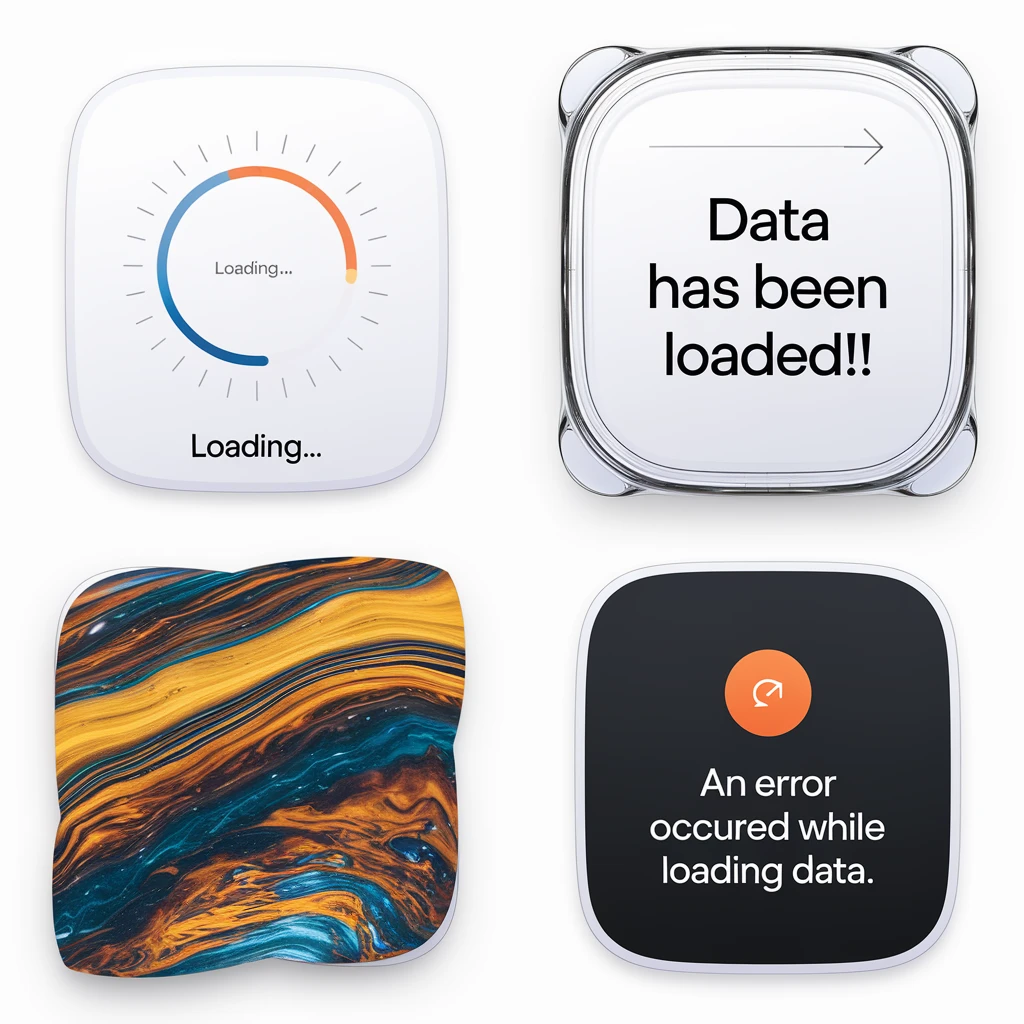
Understanding Loading States in Livewire
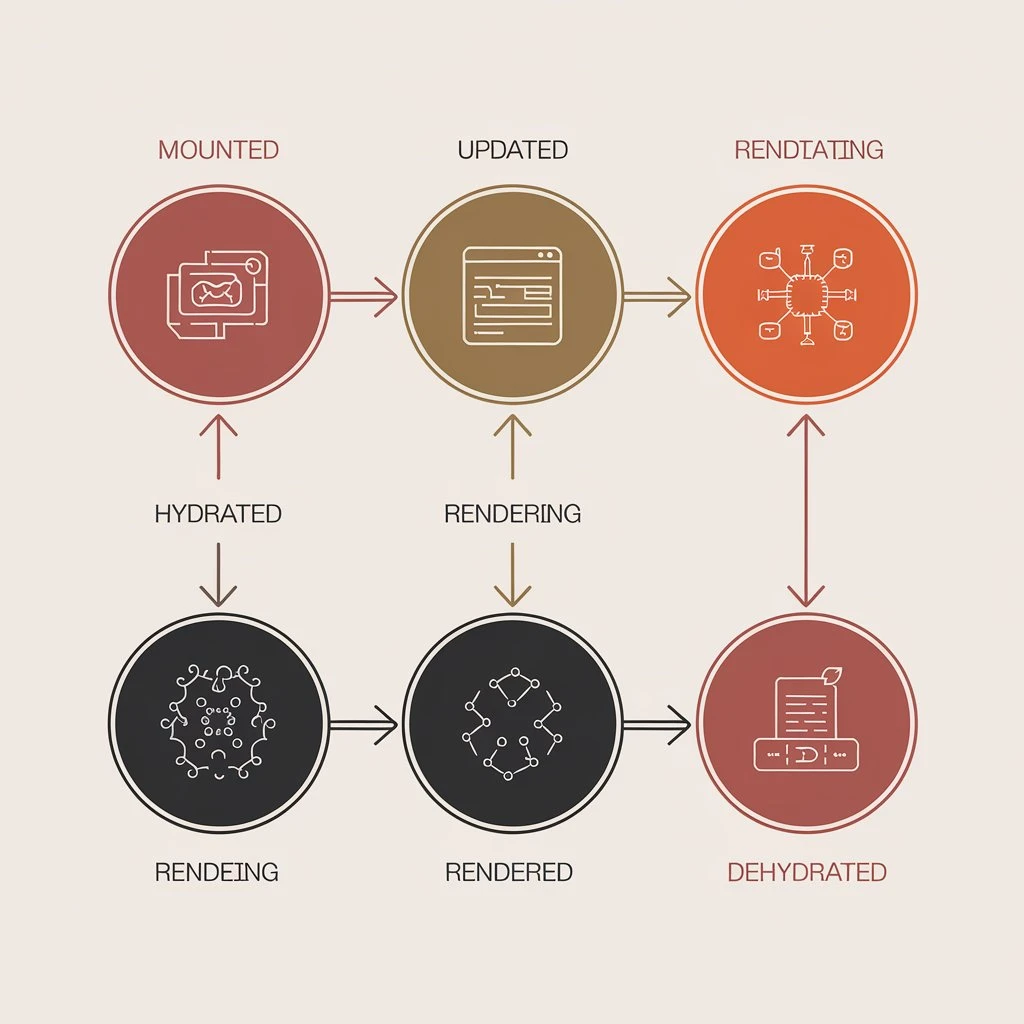
Understanding Lifecycle Hooks in Laravel Livewire
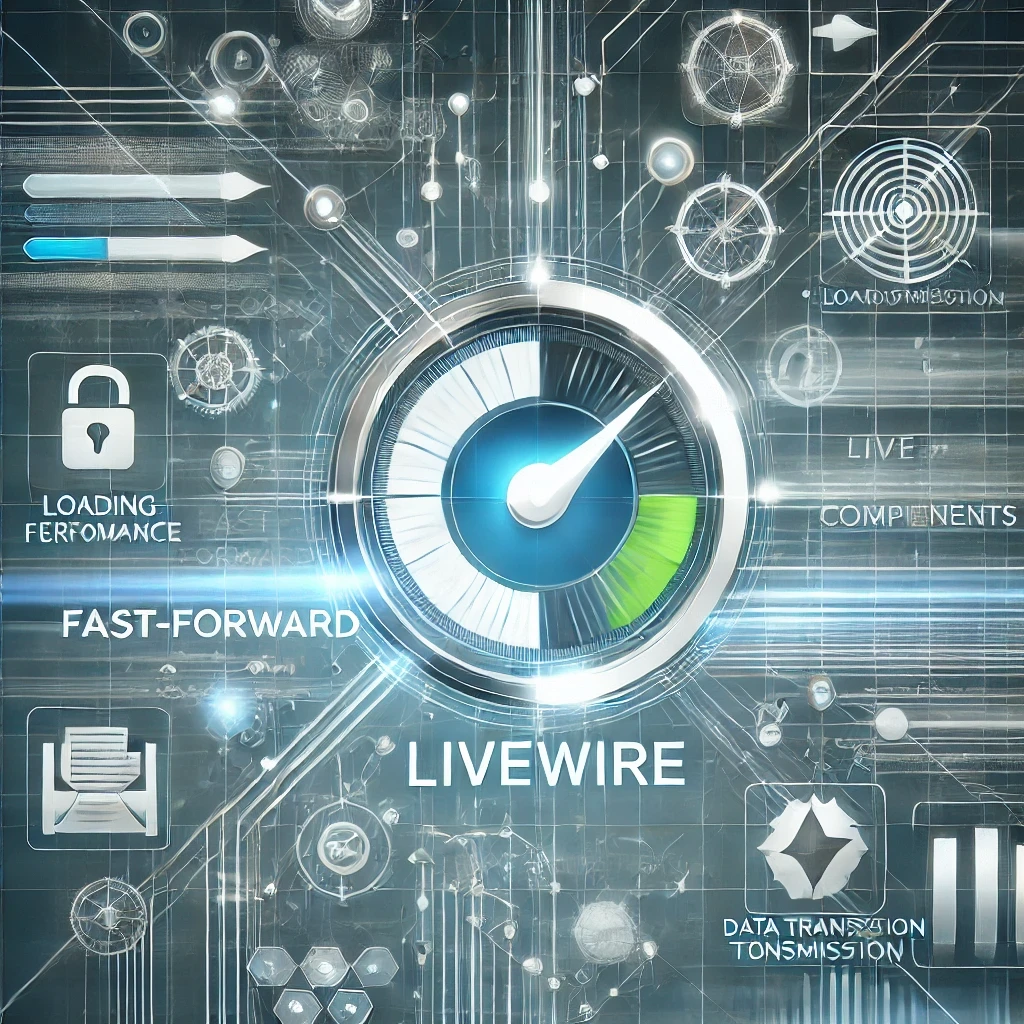
Optimizing Livewire Performance for Faster Web Applications
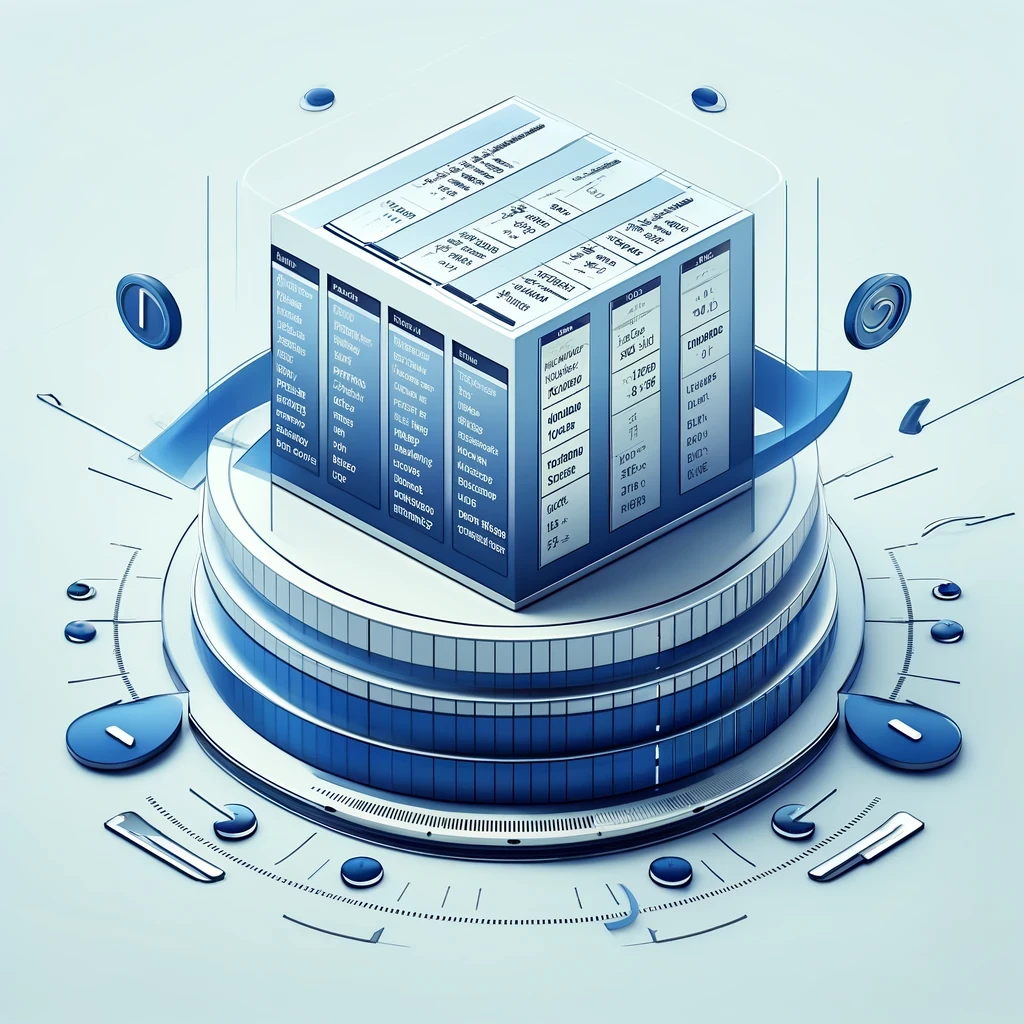