- Data Binding in Livewire A Seamless Way to Sync Data
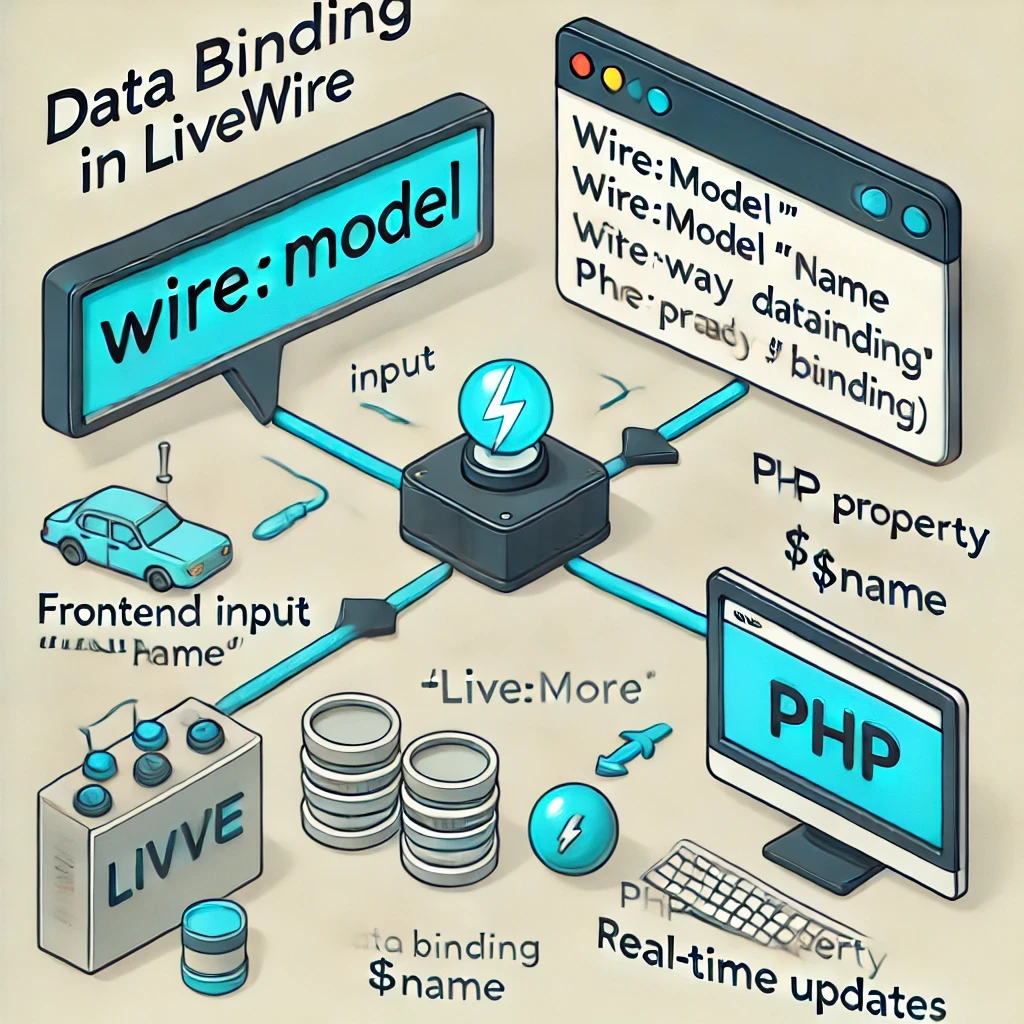
- 6 months ago
- 6 min read
Data binding is one of the key features that makes Livewire a powerful tool for Laravel developers. It enables effortless synchronization between your application's frontend and backend, without requiring any JavaScript. With Livewire, data binding ensures that changes in your user interface are automatically reflected in the underlying data and vice versa. This feature simplifies the development of dynamic and interactive applications, keeping everything in PHP.
What is Data Binding?
Data binding is the process of linking a variable in your server-side PHP code with the corresponding value in your HTML markup. Whenever the value of the bound variable changes, Livewire automatically updates the DOM to reflect this change, and vice versa. This two-way data binding makes it easy to create dynamic, interactive applications without the need for extensive front-end logic.
In simpler terms, data binding allows your UI to stay in sync with your data model, eliminating the need to manually manage updates between them.
How Data Binding Works in Livewire
In Livewire, data binding is extremely simple. Let’s look at an example of a text input field bound to a property in a Livewire component.
- Create a Livewire Component
First, we need to create a component with a property that we want to bind to the input field.
class ExampleComponent extends Component { public $name = ''; public function render() { return view('livewire.example-component'); } }
In this example, the $name property will be bound to the text input field in the view.
- Bind the Data in the View
In the component's Blade view, we use the wire:model directive to bind the input field to the $name property.
<div> <input type="text" wire:model="name"> <p>Hello, {{ $name }}</p> </div>
Here’s what happens:
- As the user types in the input field, the value of the $name property is updated automatically.
- The paragraph (<p>Hello, {{ $name }}</p>) will dynamically update with the value of the $name property as the user types.
This two-way data binding ensures that any changes made to the UI are instantly reflected in the backend, and any updates from the backend are reflected in the UI.
Types of Data Binding in Livewire
- Text Binding
- As seen in the example, text inputs are commonly bound to Livewire properties using wire:model. This is useful for forms, search bars, or any other input fields where real-time updates are needed.
- Checkbox and Radio Button Binding
- Checkboxes and radio buttons can also be bound using wire:model. This enables instant updates based on user selections.
<input type="checkbox" wire:model="isChecked">
- In this example, the $isChecked property is updated in real-time based on the checkbox’s state.
- Dropdown Binding
- Dropdown (select) fields can also be easily bound to Livewire properties.
<select wire:model="selectedOption"> <option value="1">Option 1</option> <option value="2">Option 2</option> </select>
- Here, $selectedOption is updated whenever the user selects a new option from the dropdown.
Benefits of Data Binding in Livewire
- No Need for JavaScript
One of the most significant advantages of data binding in Livewire is that it doesn’t require you to write any JavaScript code. All the interactions are handled via PHP, allowing developers to focus entirely on the backend without worrying about frontend complexities. - Instant UI Updates
Data binding in Livewire ensures that user inputs are immediately reflected in the user interface. This creates a seamless experience for the end user, as changes to data are instantly visible without requiring a page reload. - Cleaner Codebase
With Livewire’s data binding, you can achieve complex interactivity with minimal code. There’s no need to manually handle event listeners, AJAX calls, or DOM updates. This results in a cleaner and more maintainable codebase. - Tight Integration with Laravel
Livewire’s data binding is deeply integrated with Laravel’s ecosystem. This means you can use features like validation, relationships, and eloquent models in your Livewire components, making it easy to manage your application’s data flow.
Debouncing and Lazy Binding
Livewire also offers ways to optimize data binding performance:
- Debouncing:
By default, every keystroke in an input field triggers a request to update the bound property. This can be optimized using debouncing to wait for the user to finish typing before sending the request. <input type="text" wire:model.debounce.500ms="name">
- In this case, Livewire will wait for 500 milliseconds of inactivity before updating the $name property.
- Lazy Binding:
If you only want the data to be updated when the input field loses focus (rather than on every keystroke), you can use the wire:model.lazy directive. <input type="text" wire:model.lazy="name">
- This approach improves performance by reducing the number of requests sent to the server.
Use Cases for Data Binding in Livewire
- Forms:
Data binding is ideal for form handling. Input fields, checkboxes, and dropdowns can be bound to Livewire properties to keep the form’s state in sync with the backend. - Search Fields:
Real-time search results can be implemented effortlessly by binding a search input to a Livewire property and updating the results as the user types. - Interactive UI Components:
Any part of your UI that requires dynamic updates, such as shopping carts, counters, or filtering options, can be built with data binding.
Conclusion
Data binding in Livewire provides Laravel developers with a powerful and efficient way to build dynamic applications without relying on JavaScript. With simple, declarative syntax, Livewire enables two-way data synchronization between the frontend and backend, allowing you to build modern, interactive user interfaces while focusing solely on PHP.
Whether you’re handling form submissions, building search functionality, or creating interactive UI components, Livewire’s data binding will simplify your development process, helping you create dynamic applications with ease.
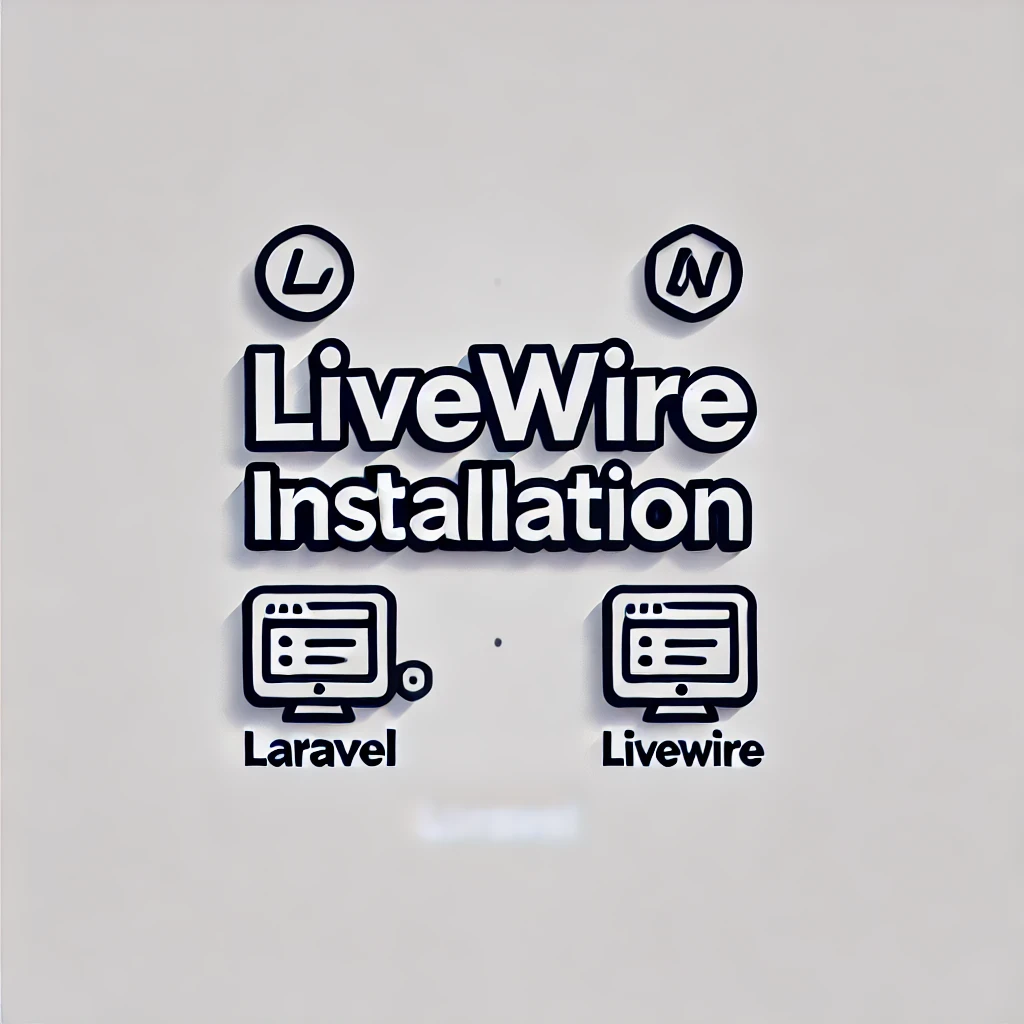
Step-by-step tutorial to install Livewire
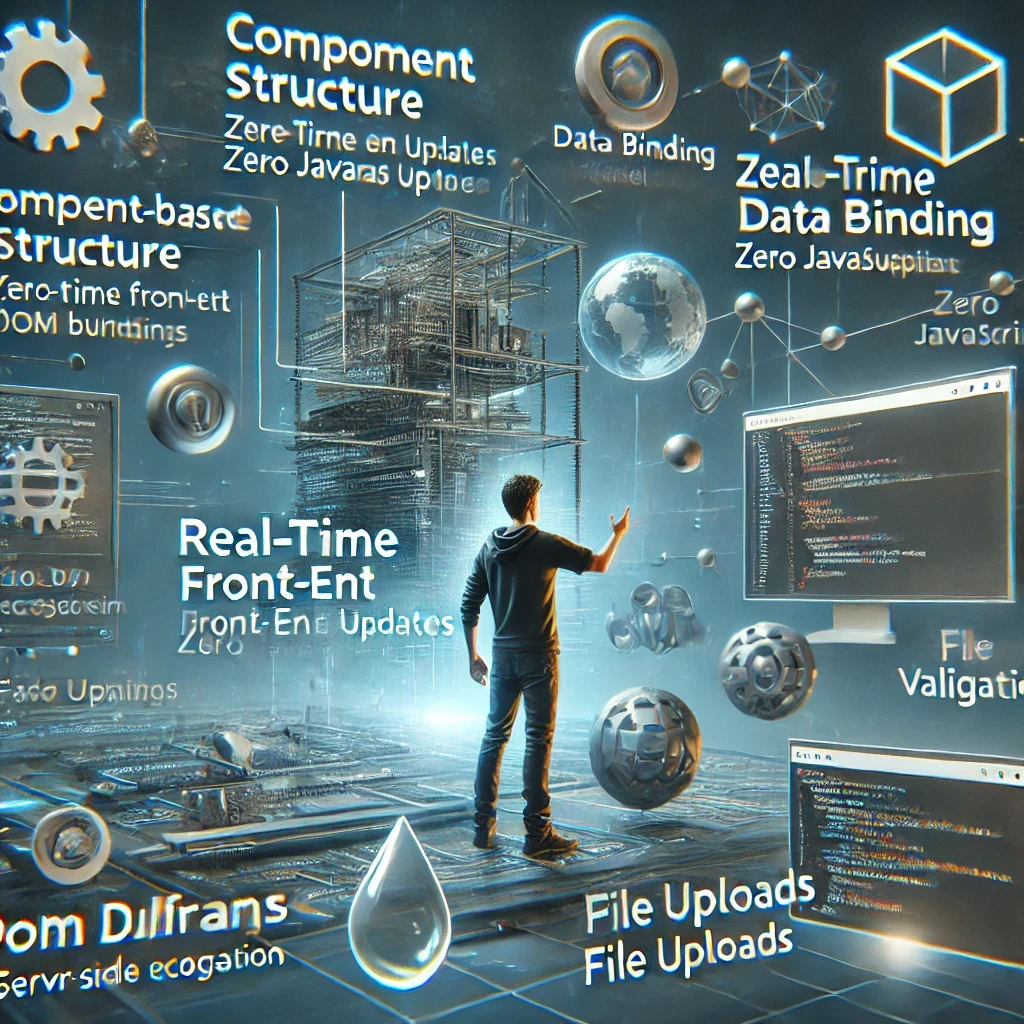
Livewire Key Features That Make It Stand Out
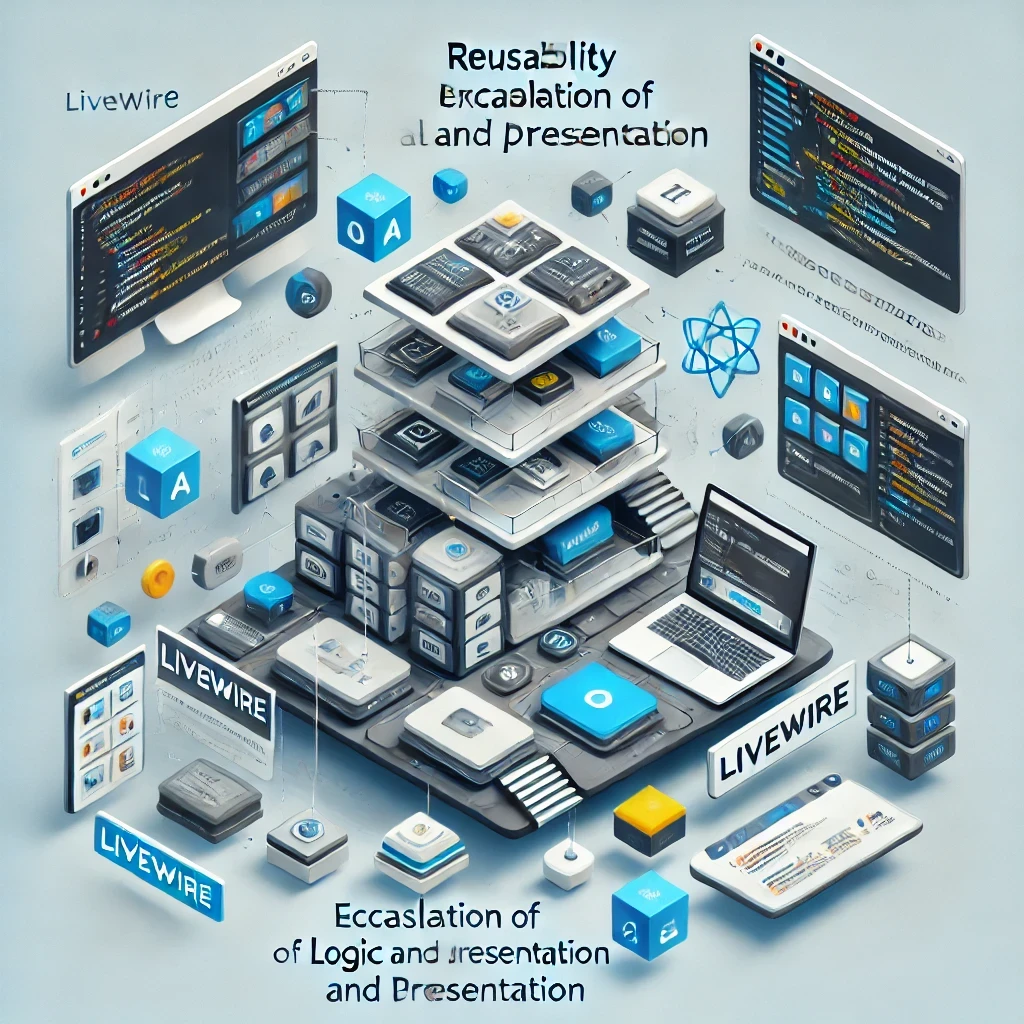
What is Component-Based Architecture ?
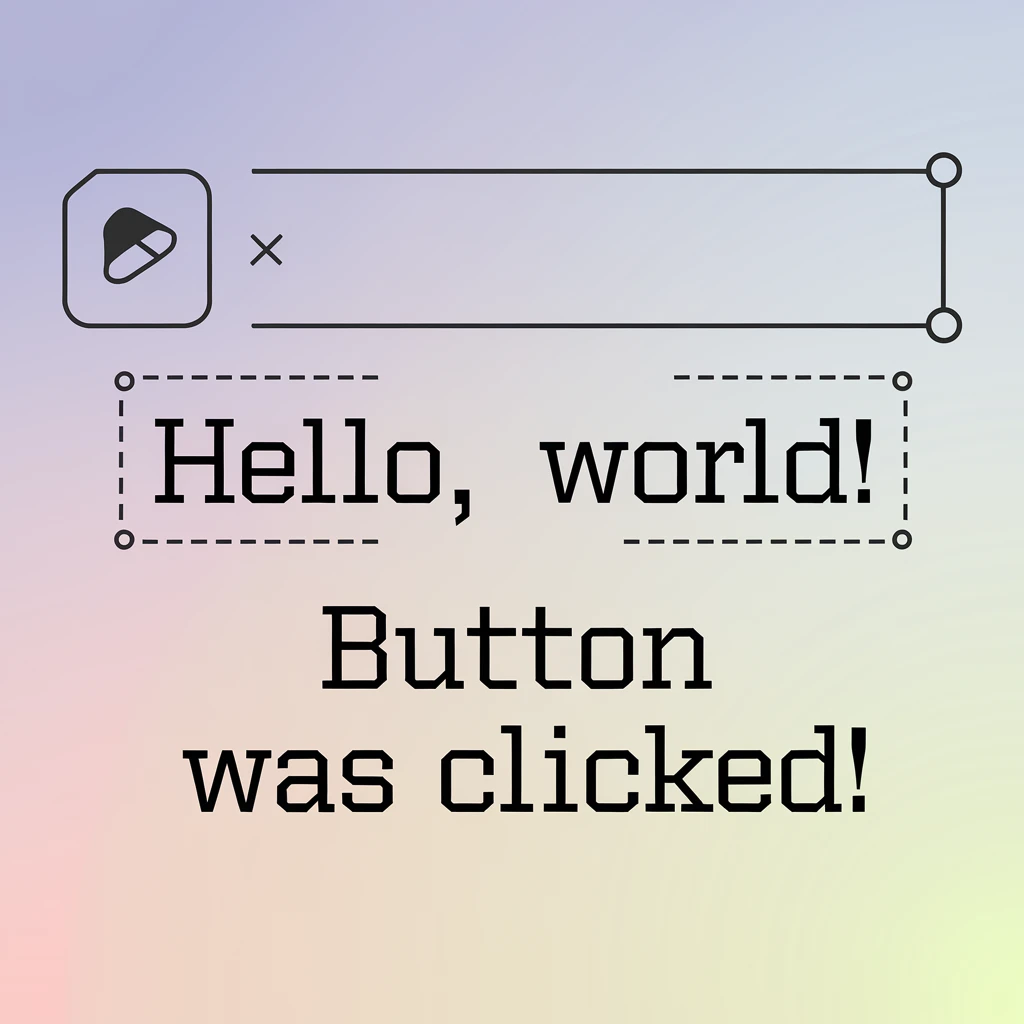
Real-Time Front-End Updates in Livewire
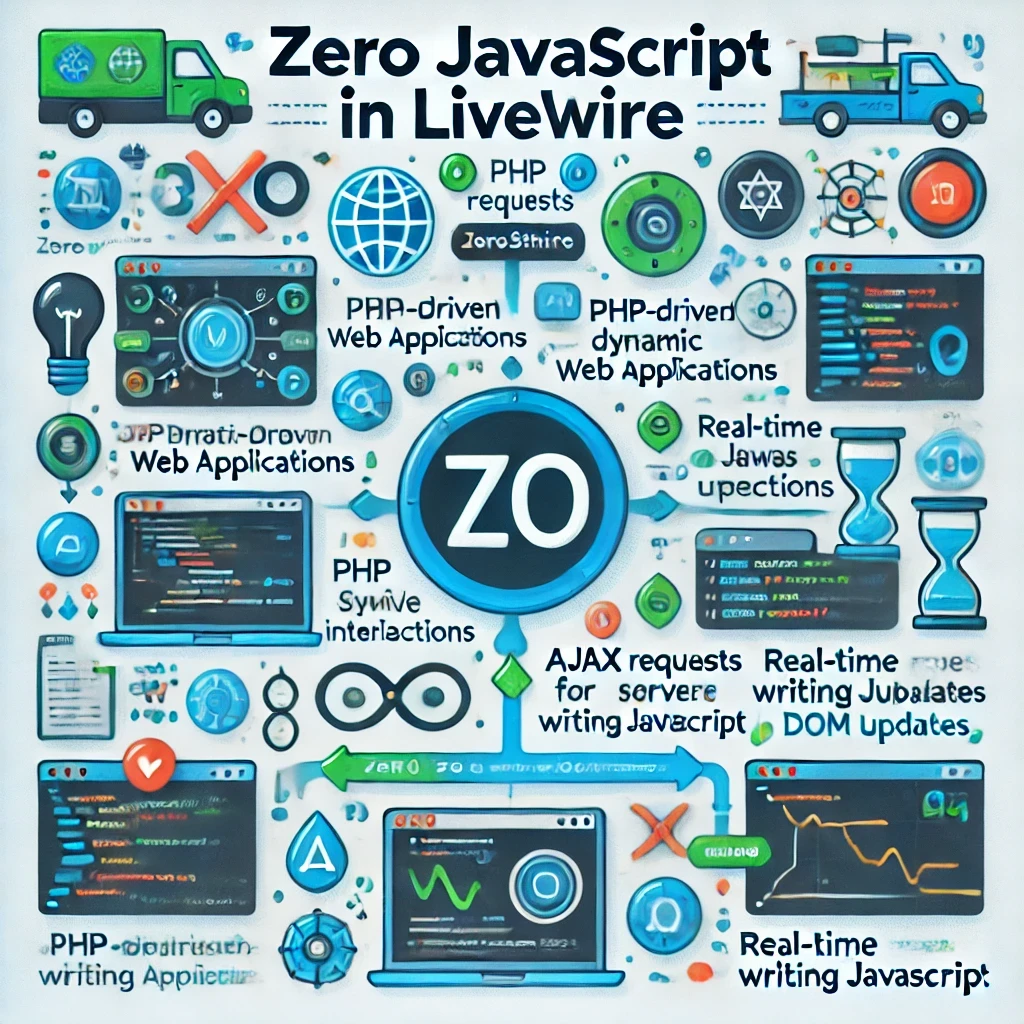
Zero JavaScript in Livewire Revolutionizing Laravel Development
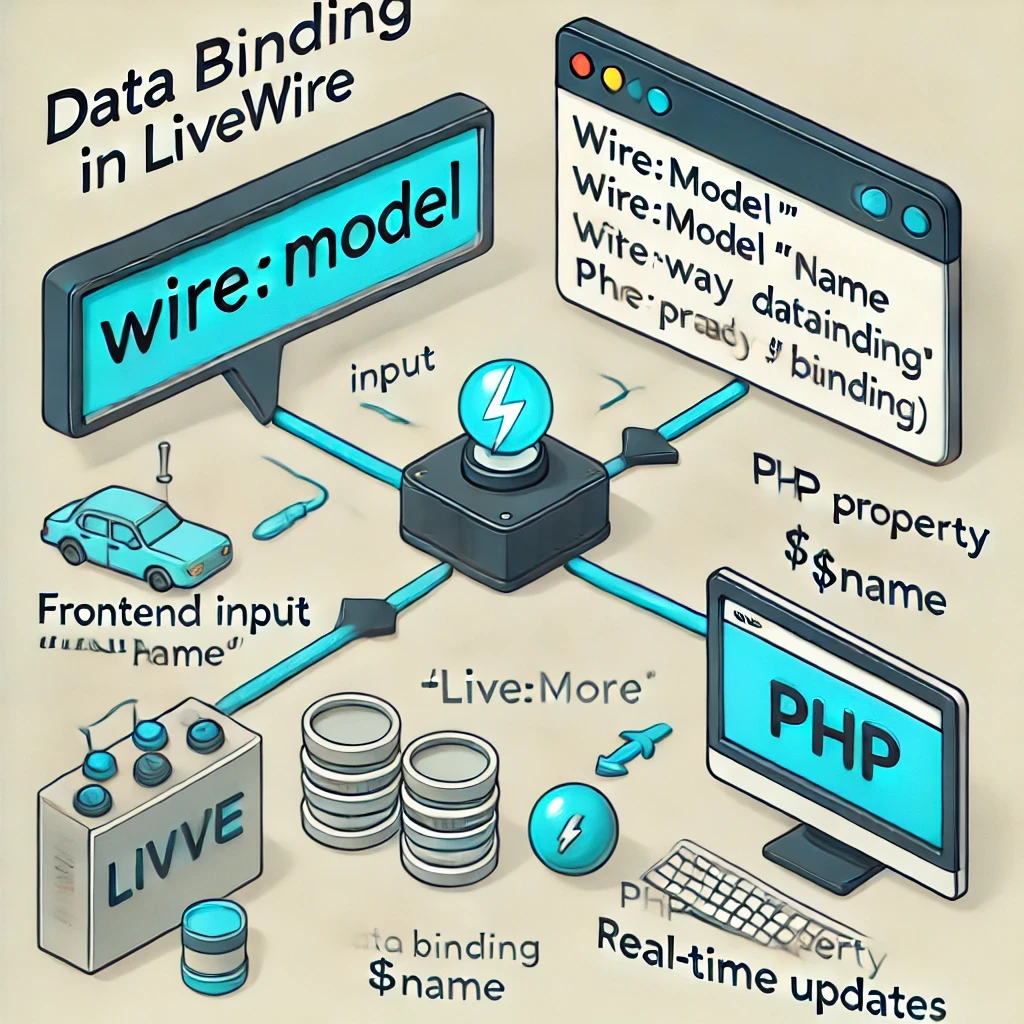
Data Binding in Livewire A Seamless Way to Sync Data
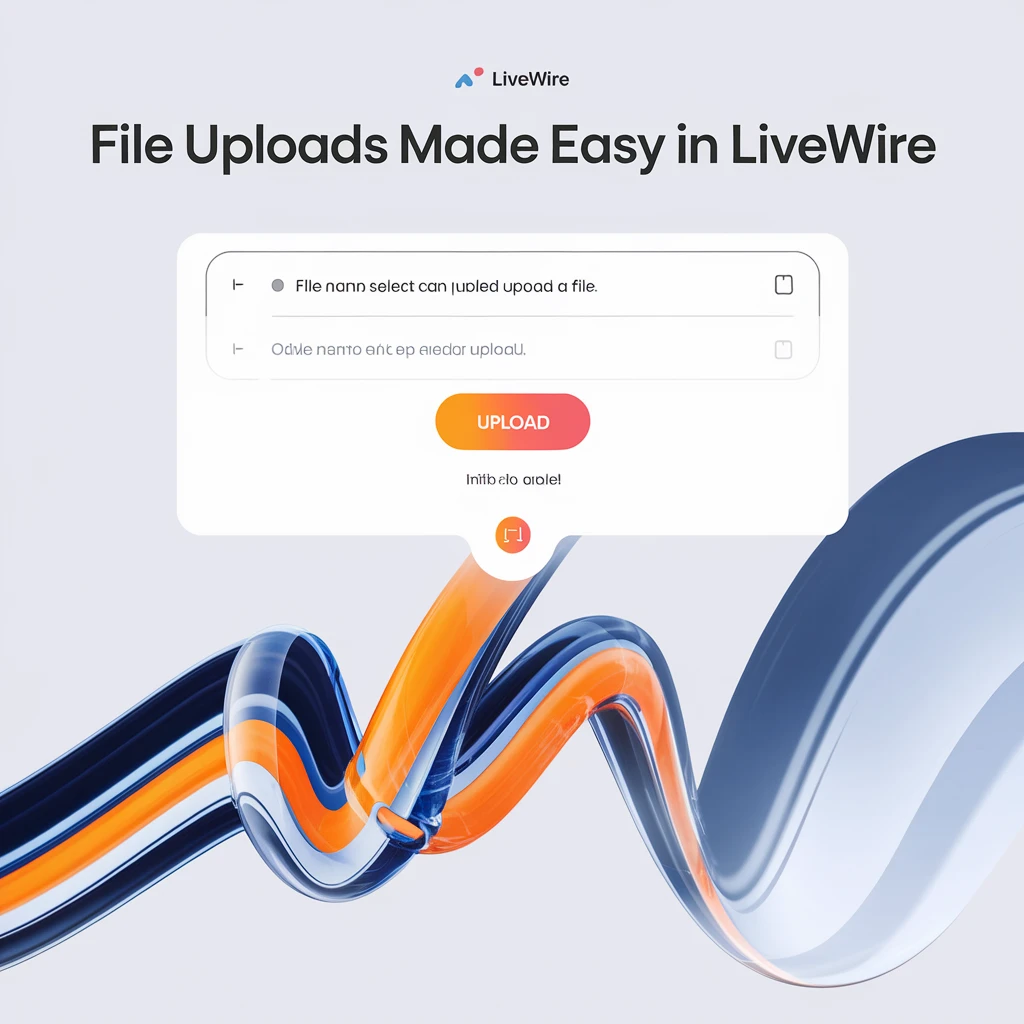
File Uploads Made Easy in Livewire
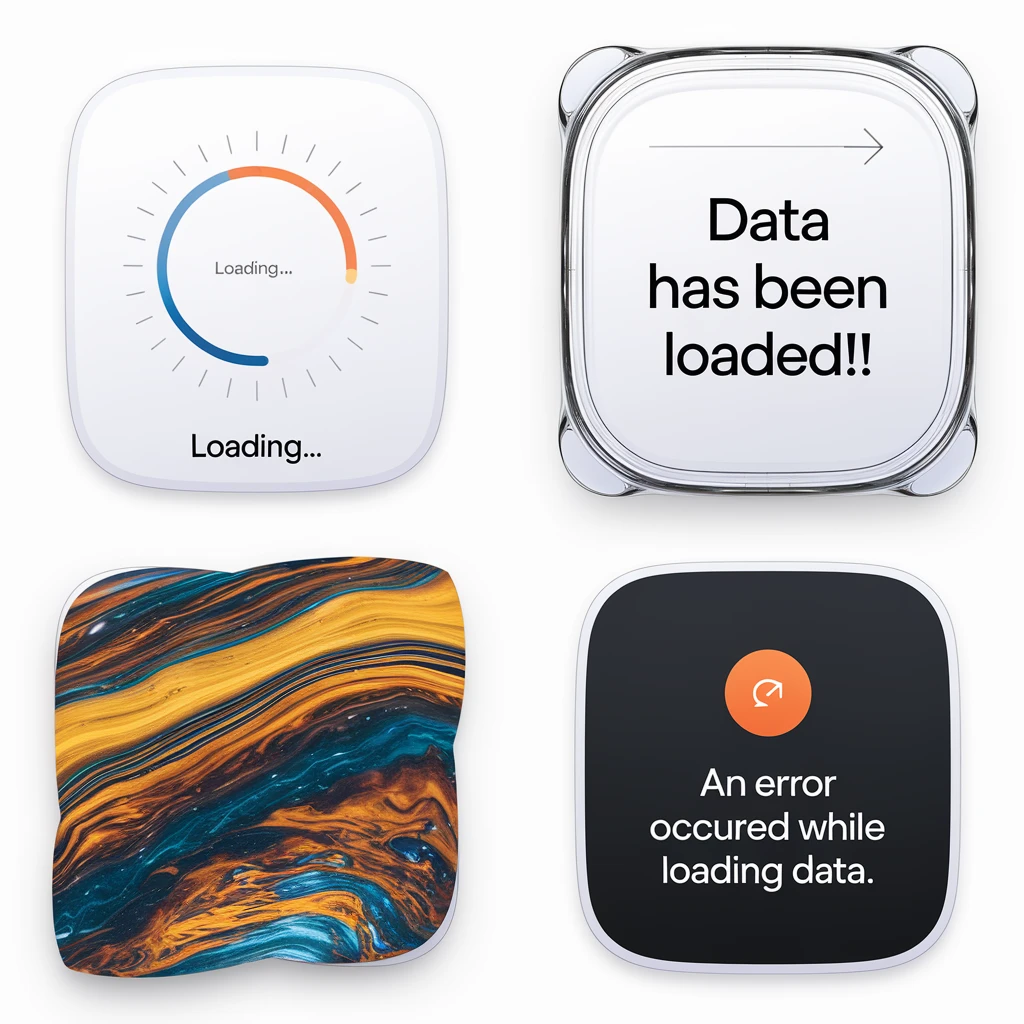
Understanding Loading States in Livewire
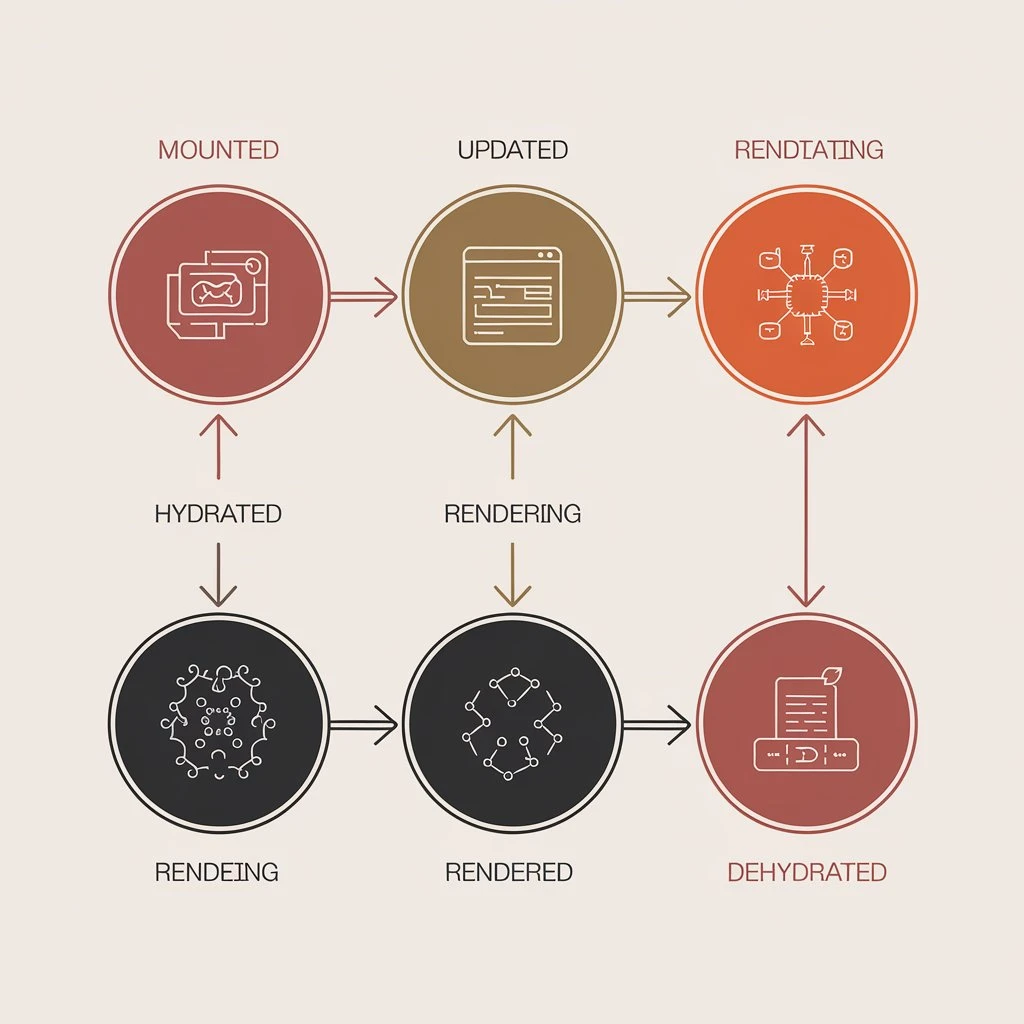
Understanding Lifecycle Hooks in Laravel Livewire
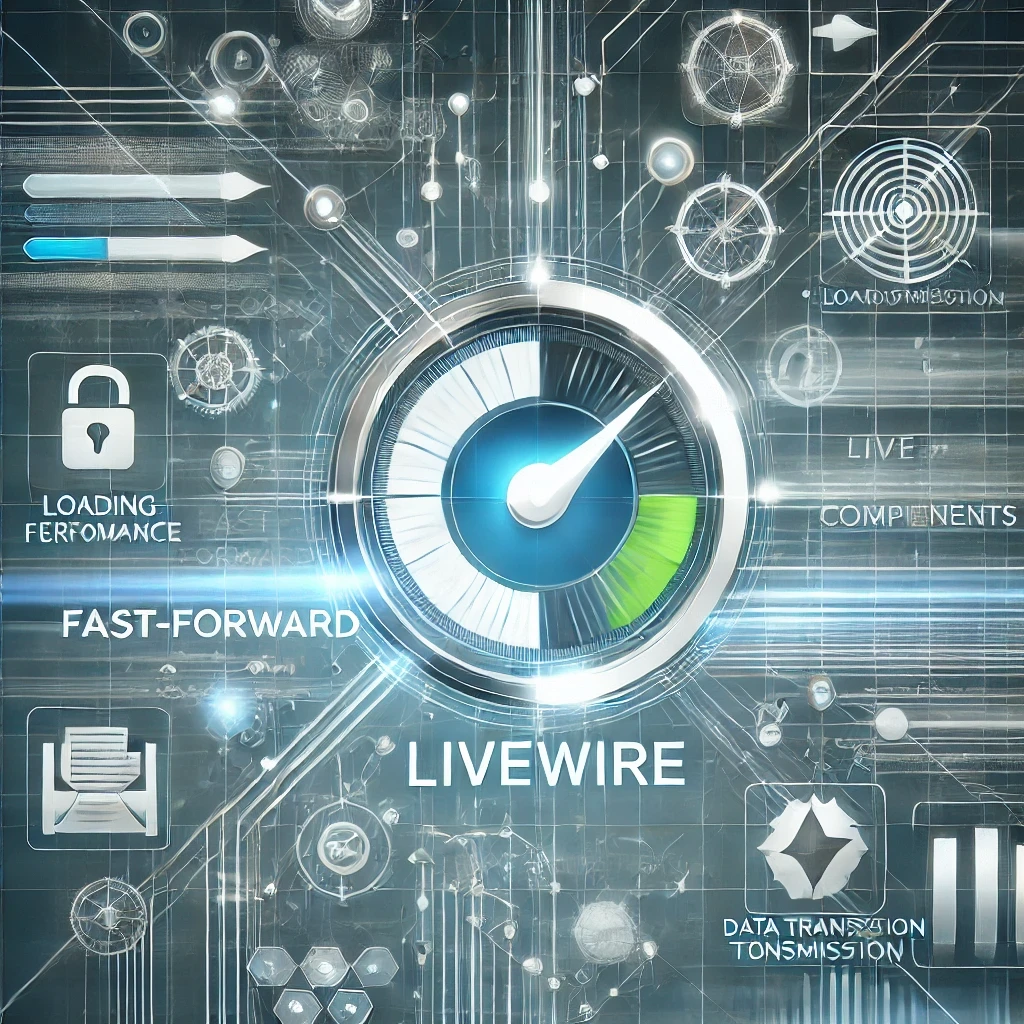
Optimizing Livewire Performance for Faster Web Applications
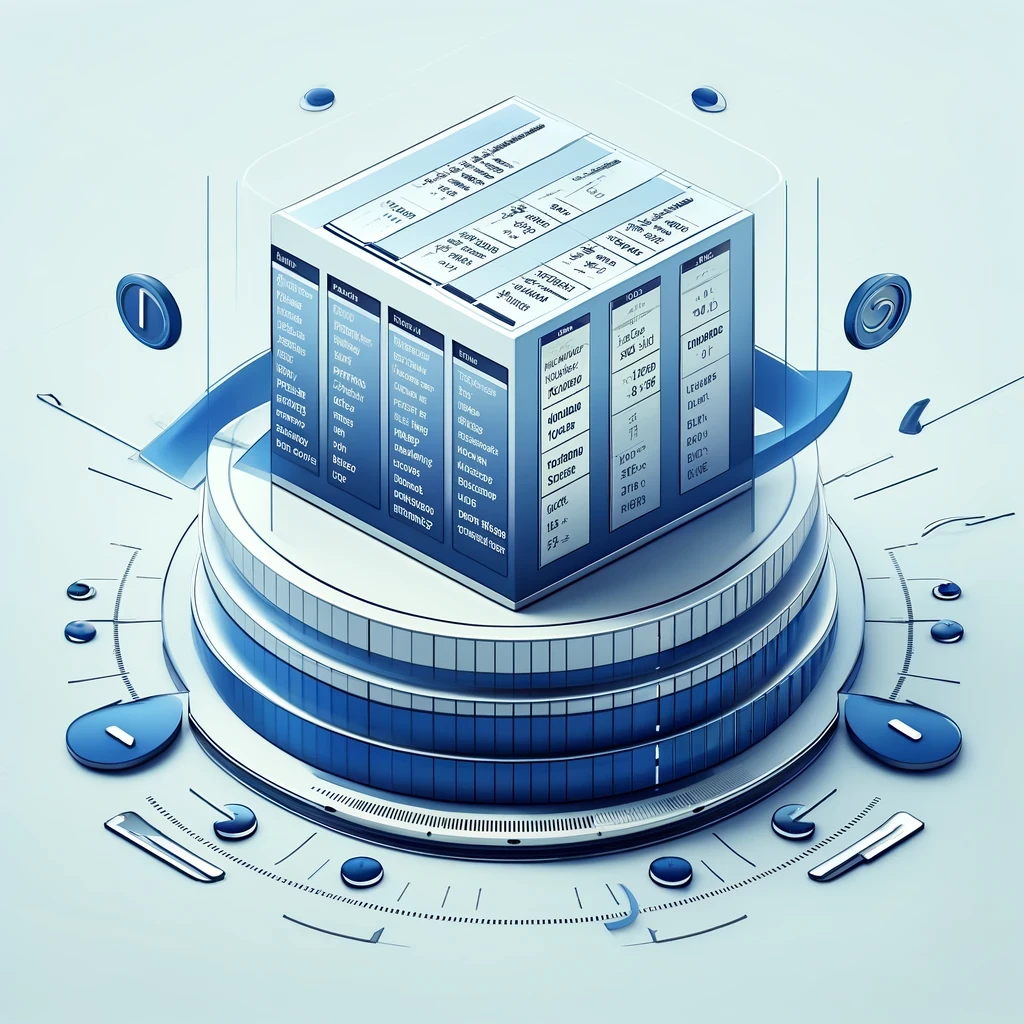